Console ExampleΒΆ
This example shows custom functions used to write to the Console in different colors.
build.cmd
:
@echo off
cd %~dp0
SETLOCAL
SET NUGET_VERSION=latest
SET CACHED_NUGET=%LocalAppData%\NuGet\nuget.%NUGET_VERSION%.exe
IF EXIST "%CACHED_NUGET%" goto copynuget
echo Downloading latest version of NuGet.exe...
IF NOT EXIST "%LocalAppData%\NuGet" md "%LocalAppData%\NuGet"
@powershell -NoProfile -ExecutionPolicy unrestricted -Command "$ProgressPreference = 'SilentlyContinue'; Invoke-WebRequest 'https://dist.nuget.org/win-x86-commandline/%NUGET_VERSION%/nuget.exe' -OutFile '%CACHED_NUGET%'"
:copynuget
IF EXIST .nuget\nuget.exe goto restore
md .nuget
copy "%CACHED_NUGET%" .nuget\nuget.exe > nul
:restore
IF EXIST packages\Sake goto run
.nuget\NuGet.exe install Sake -ExcludeVersion -Source https://www.nuget.org/api/v2/ -Out packages
:run
packages\Sake\tools\Sake.exe -I imports -f makefile.shade %*
Console.shade
saved to the imports
directory:
use namespace="System"
use namespace="System.IO"
functions
@{
void WriteLine(string text, string colorText)
{
ConsoleColor color;
if (Enum.TryParse<ConsoleColor>(colorText, true, out color))
{
WriteLine(text, color);
return;
}
WriteLine(text);
}
void WriteLine(string text = null, ConsoleColor? color = null)
{
if (text != null && color != null)
{
Console.ForegroundColor = color.Value;
}
Console.WriteLine(text);
if (text != null && color != null)
{
Console.ResetColor();
}
}
void Write(string text, string colorText)
{
ConsoleColor color;
if (Enum.TryParse<ConsoleColor>(colorText, true, out color))
{
Write(text, color);
return;
}
Write(text);
}
void Write(string text = null, ConsoleColor? color = null)
{
if (text != null && color != null)
{
Console.ForegroundColor = color.Value;
}
Console.Write(text);
if (text != null && color != null)
{
Console.ResetColor();
}
}
}
makefile.shade
:
use namespace="System.Linq"
use import="Console"
#default
@{
WriteLine();
WriteLine(" Colors in ConsoleColor", "yellow");
WriteLine(" ======================", "cyan");
foreach(var color in Enum.GetValues(typeof(ConsoleColor)).Cast<ConsoleColor>())
{
Write(" ");
WriteLine(color.ToString(), color);
}
WriteLine();
WriteLine(" ======================", "cyan");
WriteLine();
}
Output:
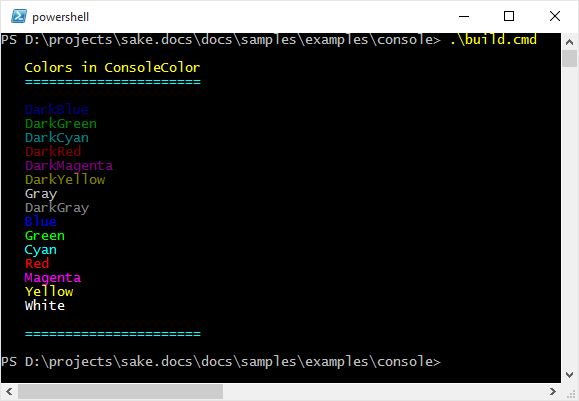